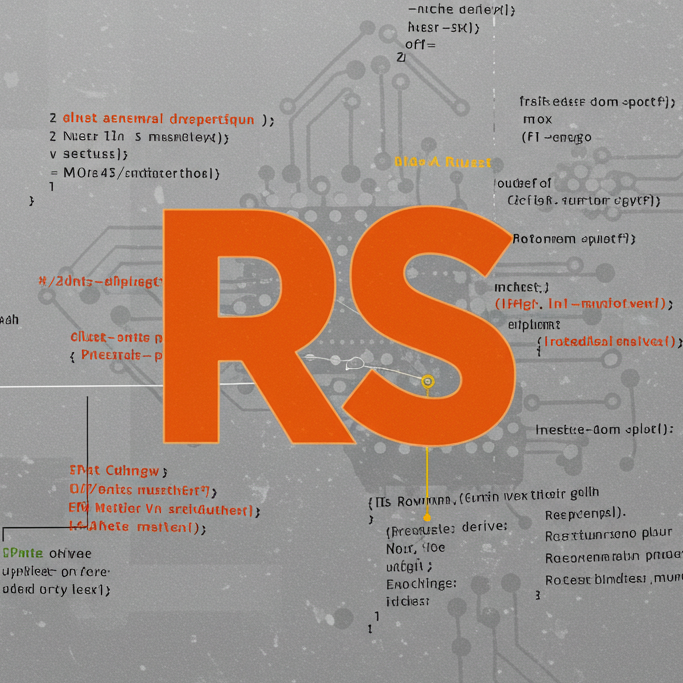
Rust is a powerful language that emphasizes safety and performance. While it doesn’t have traditional “extension methods” like some other languages, it achieves similar functionality through traits. This article will show you how to extend existing types with new behavior using traits in Rust.
Part 1: Basic String Extensions
Let’s start with a simple example. Suppose we want to add some utility functions to the String
type. We can define a trait called StringExtensions
:
trait StringExtensions {
fn is_empty(&self) -> bool;
fn is_white_space_only(&self) -> bool;
}
impl StringExtensions for String {
fn is_empty(&self) -> bool {
self.len() == 0
}
fn is_white_space_only(&self) -> bool {
self.trim().is_empty()
}
}
Explanation:
- We define a trait
StringExtensions
with two methods:is_empty
andis_white_space_only
. - We then implement this trait for the
String
type. This means we’re adding these methods to allString
instances.
Now, we can use these new methods on any String
:
fn main() {
let s = String::from(" ");
println!("Is empty: {}", s.is_empty()); // false
println!("Is whitespace only: {}", s.is_white_space_only()); // true
}
Part 2: Generic Extensions for Vectors
Traits can also be implemented for generic types. Let’s extend the Vec
type with an is_empty
method:
trait VecExtensions {
fn is_empty(&self) -> bool;
}
impl<T> VecExtensions for Vec<T> {
fn is_empty(&self) -> bool {
self.len() == 0
}
}
Explanation:
- We define a trait
VecExtensions
with anis_empty
method. - We implement this trait for
Vec<T>
, whereT
can be any type.
Now, any Vec
can use this method:
fn main() {
let v: Vec<i32> = vec![1, 2, 3];
println!("Is vector empty: {}", v.is_empty()); // false
}
Part 3: Specialized Extensions
We can also create traits that are only applicable to specific types. For example, let’s create a trait for Vec<i32>
that checks for negative numbers and calculates the sum:
trait VecExtensionsForInt {
fn has_negative(&self) -> bool;
fn sum(&self) -> i32;
}
impl VecExtensionsForInt for Vec<i32> {
fn has_negative(&self) -> bool {
self.iter().any(|num| num < &0)
}
fn sum(&self) -> i32 {
self.iter().sum()
}
}
Explanation:
- We define a trait
VecExtensionsForInt
with methodshas_negative
andsum
. - We implement this trait specifically for
Vec<i32>
.
Now, we can use these methods on Vec<i32>
:
fn main() {
let v: Vec<i32> = vec![-1, 2, -3];
println!("Has negative: {}", v.has_negative()); // true
println!("Sum: {}", v.sum()); // -2
}
Part 4: Displaying Vector Elements
Let’s combine traits with other Rust features. We’ll create a Printable
trait and implement it for Vec<T>
where T
implements the Display
trait:
use std::fmt;
trait Printable {
fn print(&self);
}
impl<T: fmt::Display> Printable for Vec<T> {
fn print(&self) {
for element in self {
println!("{}", element);
}
}
}
Explanation:
- We define a trait
Printable
with aprint
method. - We implement this trait for
Vec<T>
whereT
must implement thefmt::Display
trait.
Now, we can print any Vec
whose elements can be displayed:
fn main() {
let v: Vec<String> = vec![String::from("hello"), String::from("world")];
v.print();
}
Traits in Rust provide a flexible way to extend existing types with new functionality. They can be used with both generic and specific types, and they can be combined with other Rust features like traits bounds. This makes traits a powerful tool for building modular and reusable code in Rust.